XRB Engine


XRB Engine is a simple game engine I made for the Graphics Engine class at ESAT.
It's written in C++ using the OpenGL graphics library. I also use other libraries like ImGUI, TinyOBJLoader, GLM and others.
The engine runs on Windows, Linux and Nintendo Switch thanks to the DevKitPro library & tools.
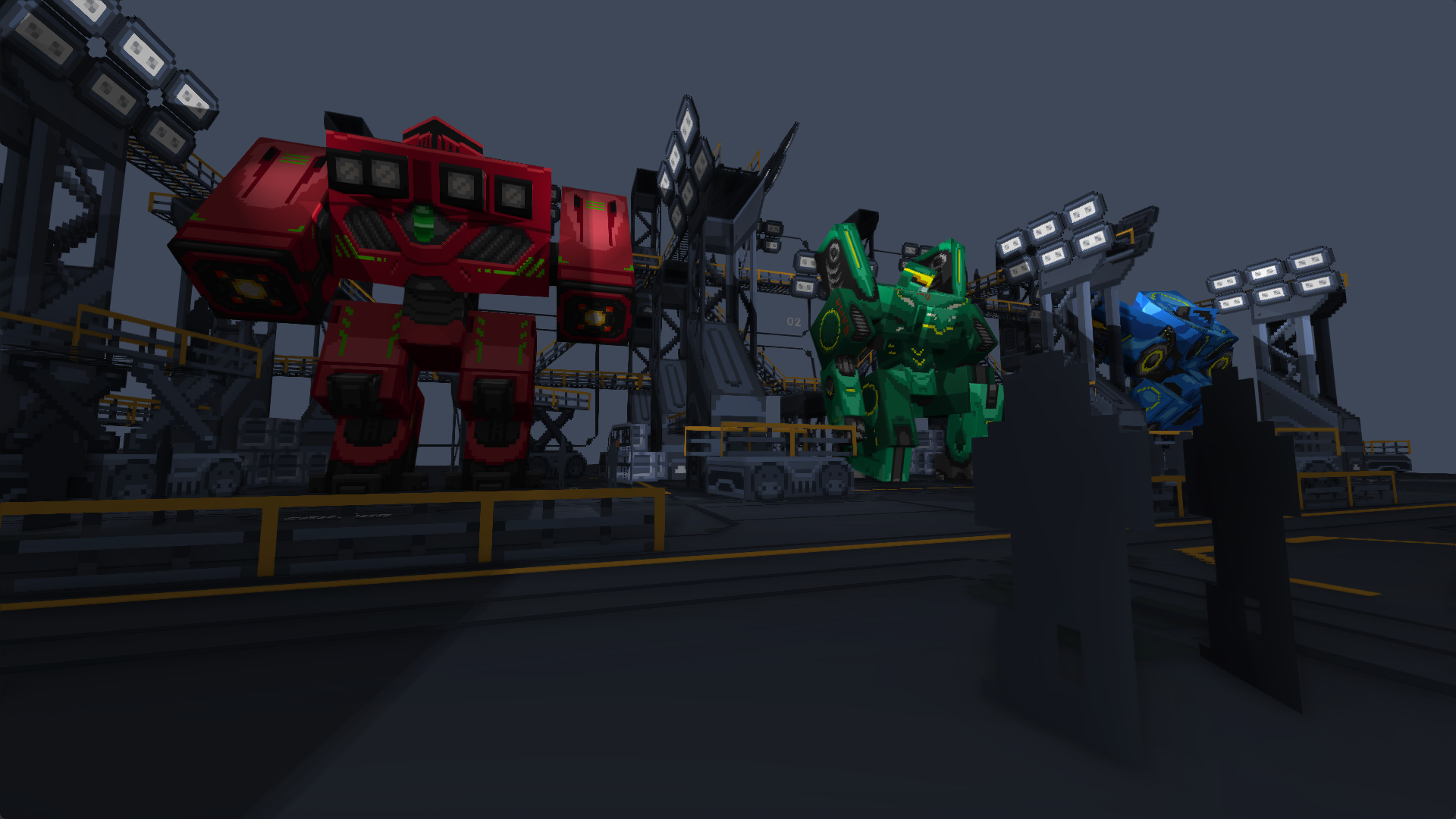
Entity Component System
An entity-component system has been integrated into the engine that allows the user to create, delete, consult and modify the different components and entities that form part of the system.
Entity l_ambient = Light::AmbientLight3D(cm, .25f, vec3(1.f)); Entity scene = cm.new_entity(); { cm.set_component<Model>(scene, mesh_scene); // Transform float x = .0f; float y = .0f; float z = .0f; Transform t{vec3(x, y, z), vec3(), vec3(1.f)}; cm.set_component<Transform>(scene, t); }
Job Manager
The Job Manager allows the engine to perform background tasks while the main activity is running on the screen, i.e. it is able to send tasks to another processor thread so as not to block the main activity. This Job Manager supports the creation of tasks which may or may not return a value.
JobSystem j_s; j_s.addTask([]() { printf("Starting simple task...\n"); for (long long i = 0; i < 50000000000; i++) ; printf("Simple task ended successfully.\n"); }); std::future<int> future_test; future_test = j_s.addTaskFuture(future_func); int future_value = future_test.get();
Forward Rendering
The engine is able to render on screen using the "Forward Rendering" method, where everything is painted on the fly.
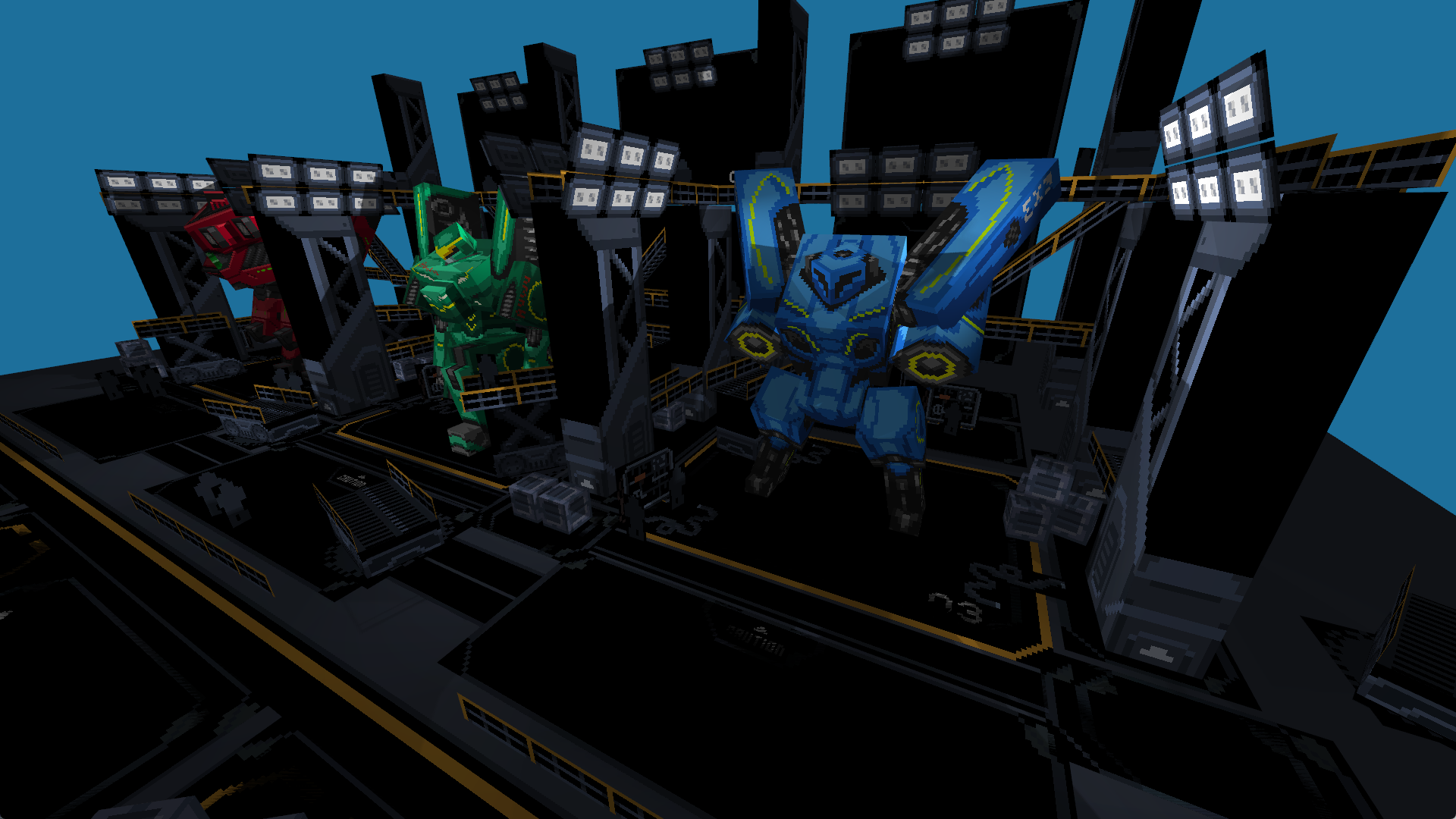
Deferred Rendering
In contrast to Forward Rendering, Deferred Rendering saves a lot of drawing calls, because in a first instance the so-called G-Buffer is filled, where in different textures the necessary information for light calculations and different effects is stored.
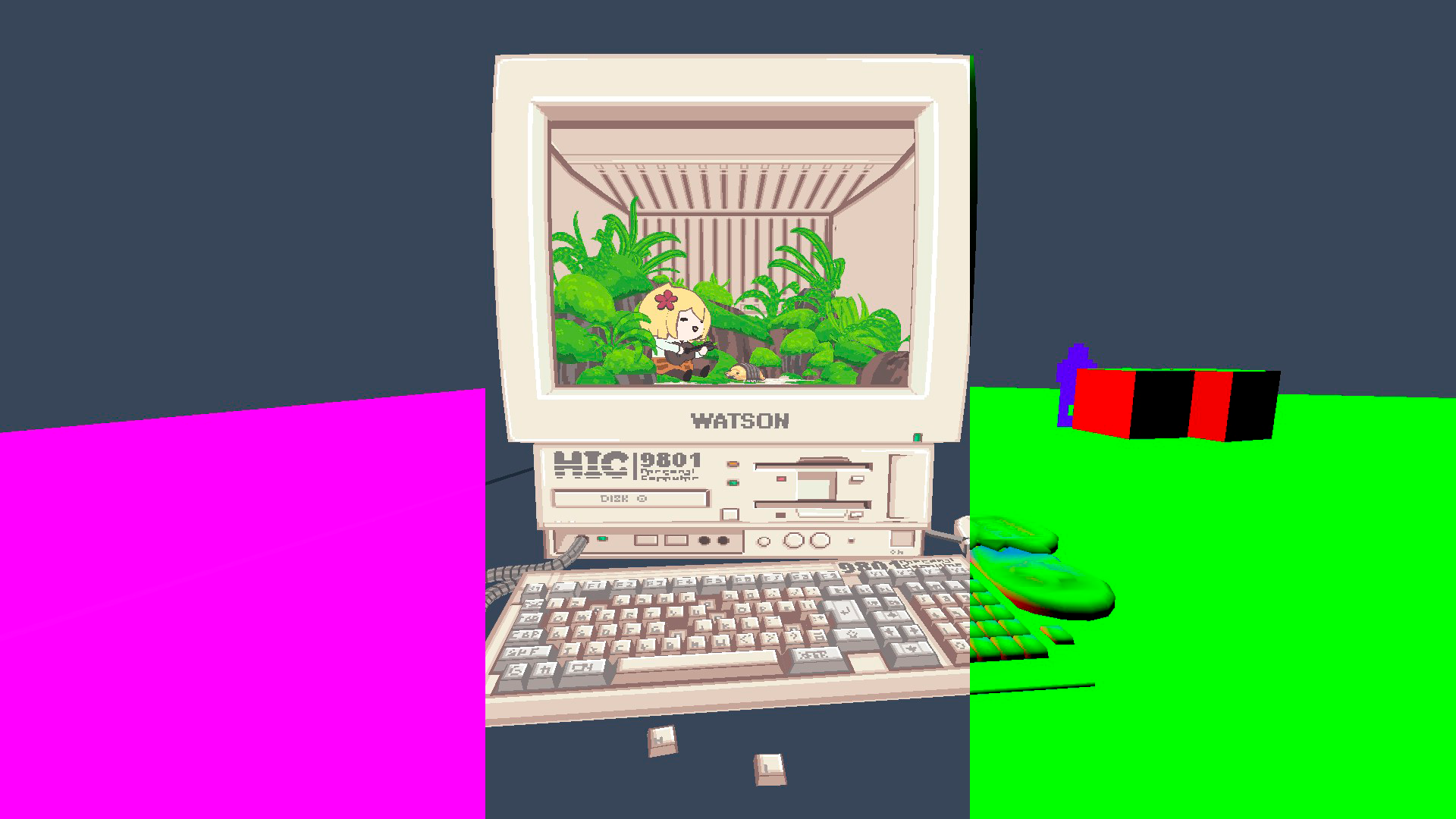
FXAA
FXAA is a post-processing effect used as an anti-aliasing solution in the Deferred Rendering method. FXAA is in charge of receiving the final texture of the screen, turning it to black and white, and with some contrast algorithms, highlighting the edges and sawtooths to apply a blur and thus apply the anti-aliasing effect. It is a very fast process that gives very good results.
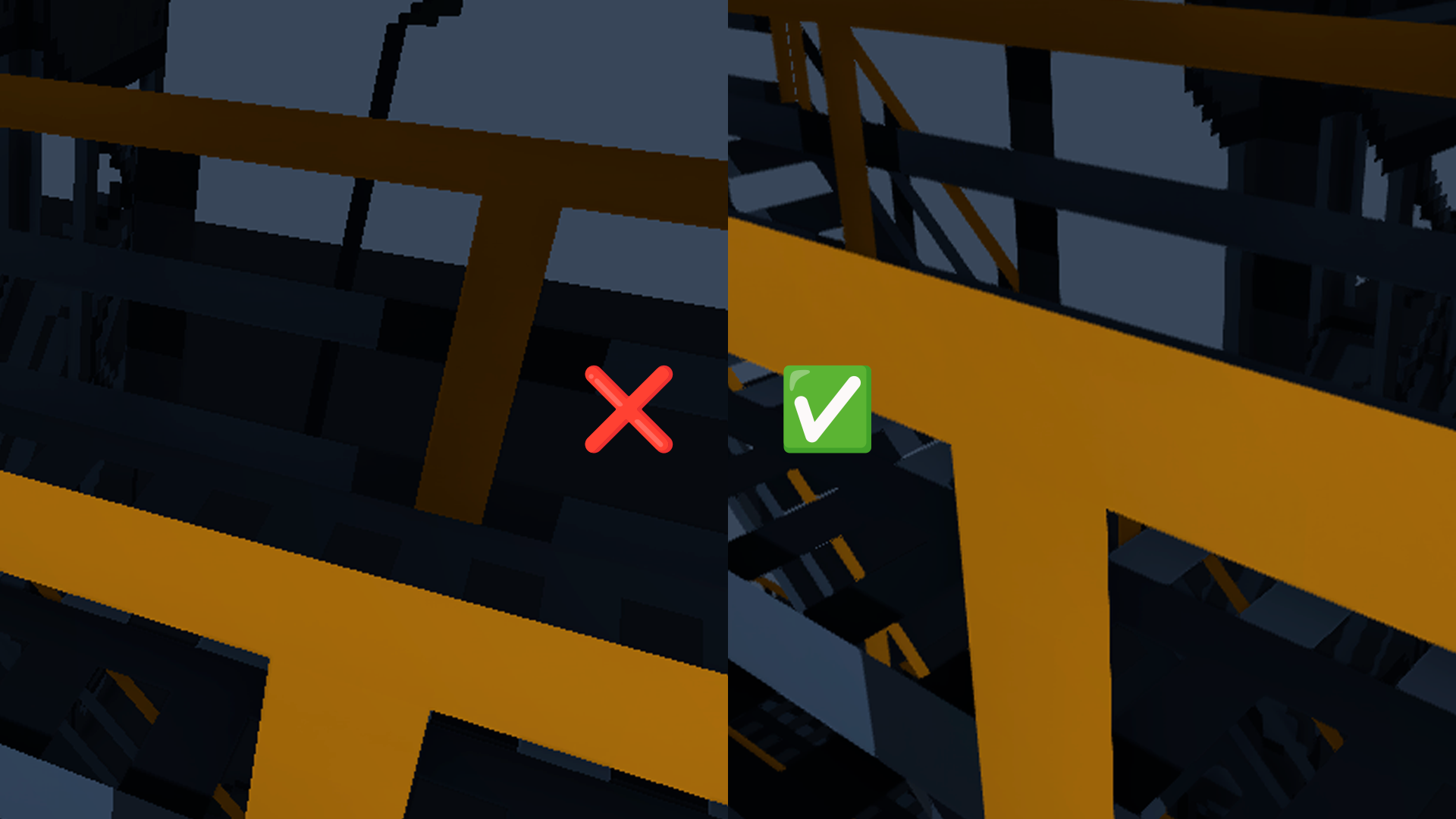
SSAO
Ambient occlusion in screen space is a graphical technique used to give a sense of volume and depth to the scene being rendered. Thanks to the use of a kernel of rotations and a simple noise texture, we can generate this texture, which after passing through a blur effect, gives a very good result.
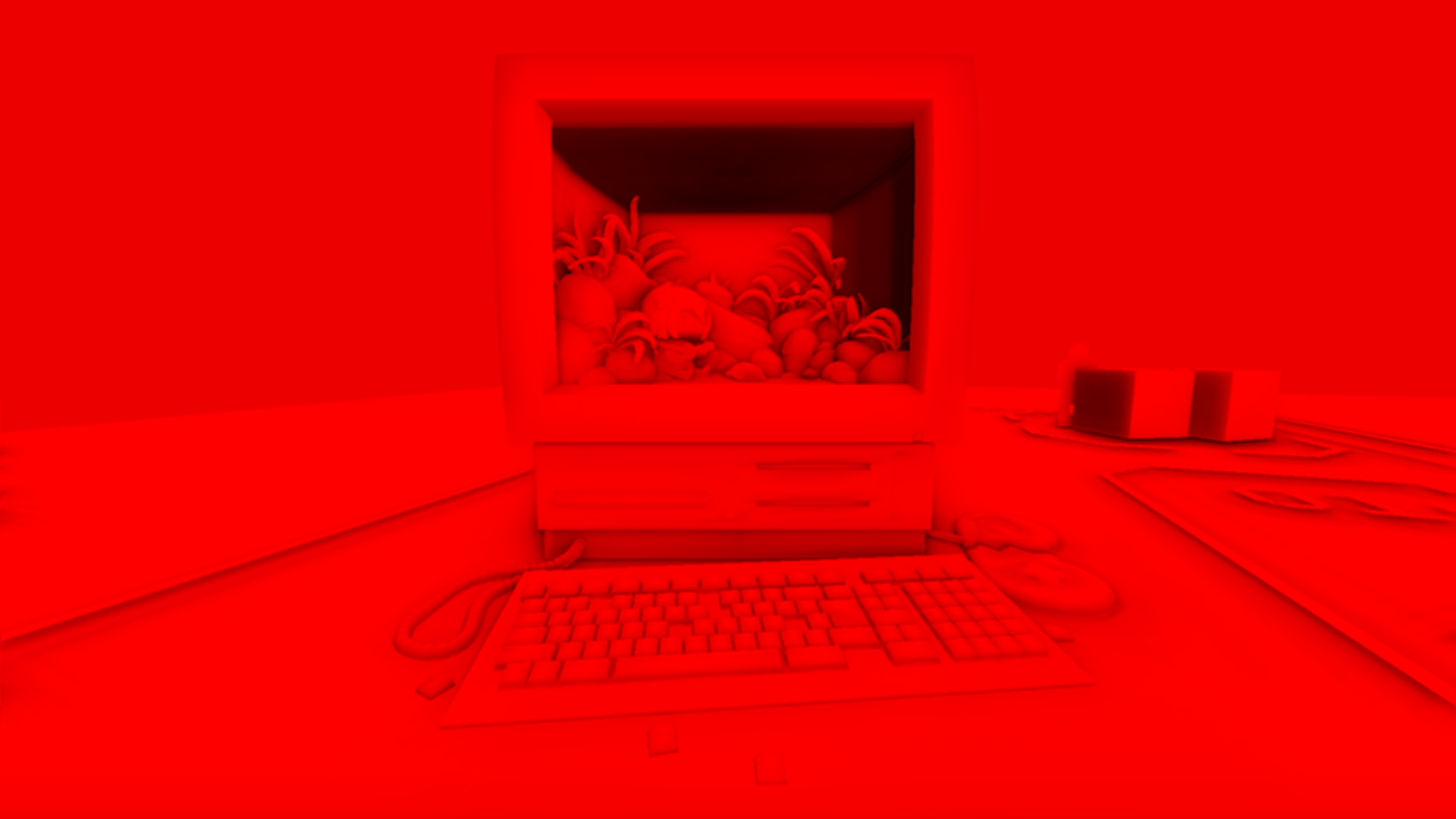
Directional Light
Directional light that illuminates the scene with a given colour and direction. The shadow it generates moves with the camera so that it does not stop painting new shadows wherever we move.
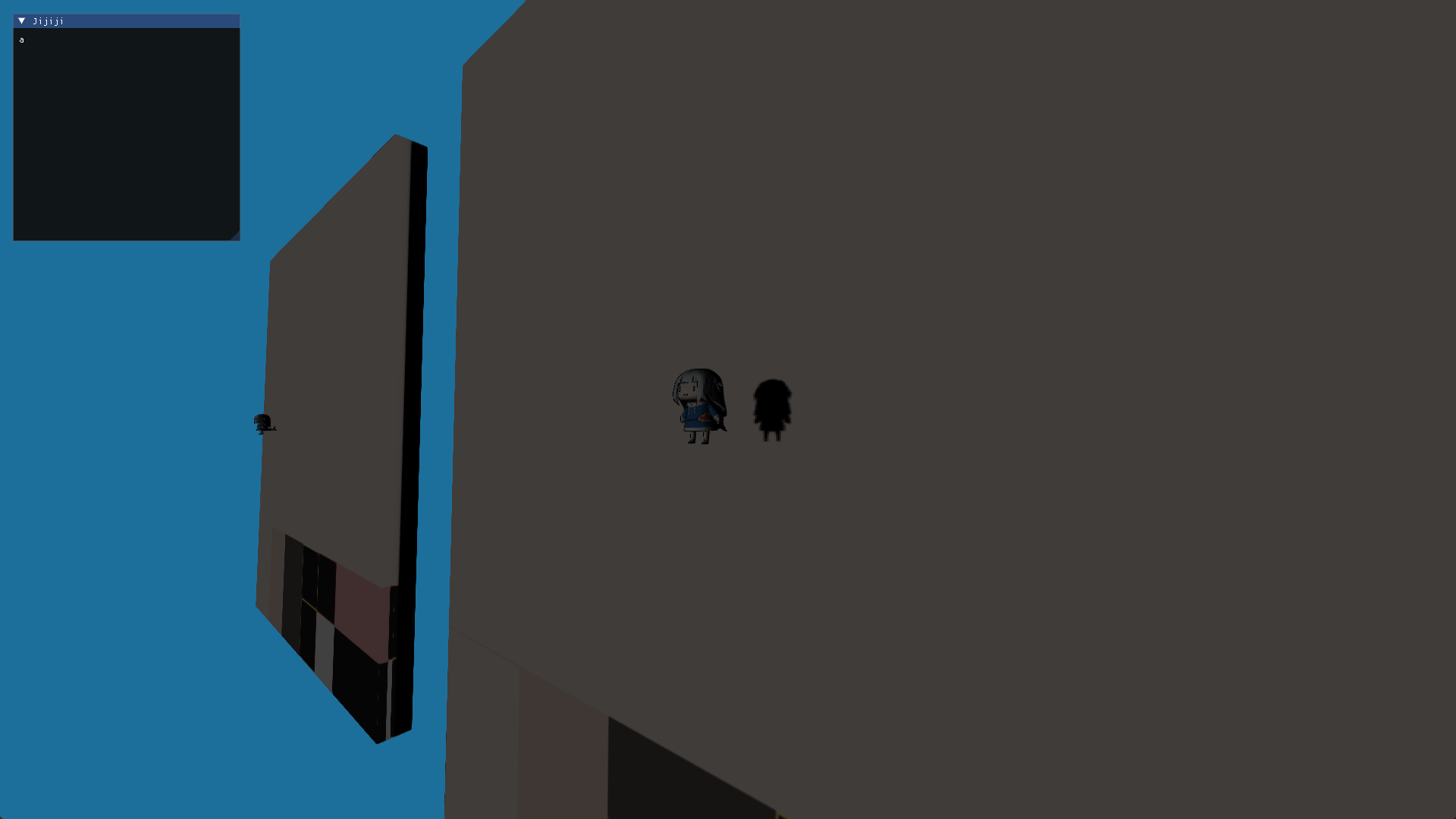
Spot Light
The spot light is a light that simulates a spotlight which generates a circular illuminated area wherever it is pointed. It also generates shadows of the objects in front of it.
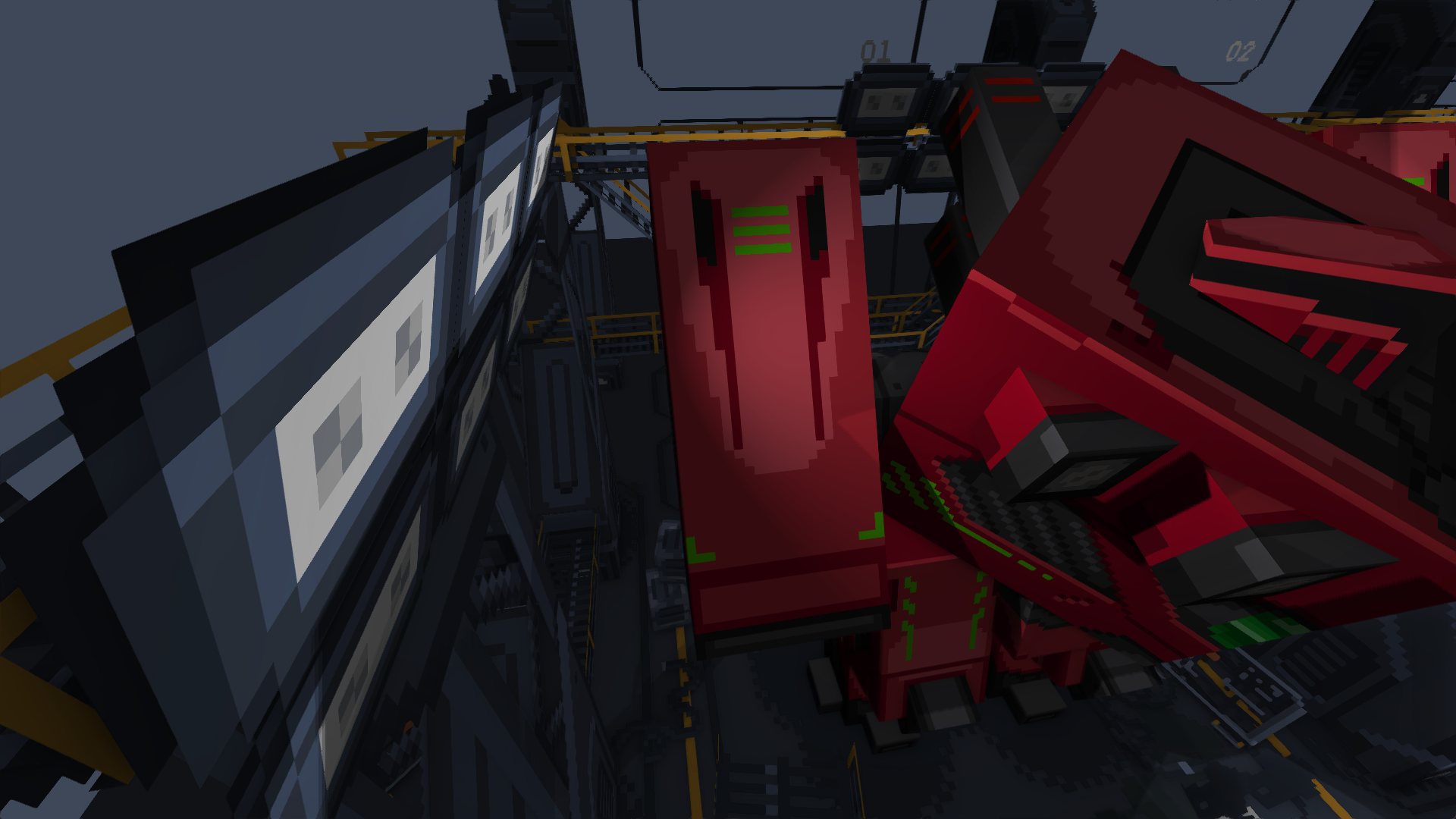
Point Light
The point light is a ball of light that shines in all directions. To generate its shadow, a cubemap is used to cast its shadow on the surrounding objects.
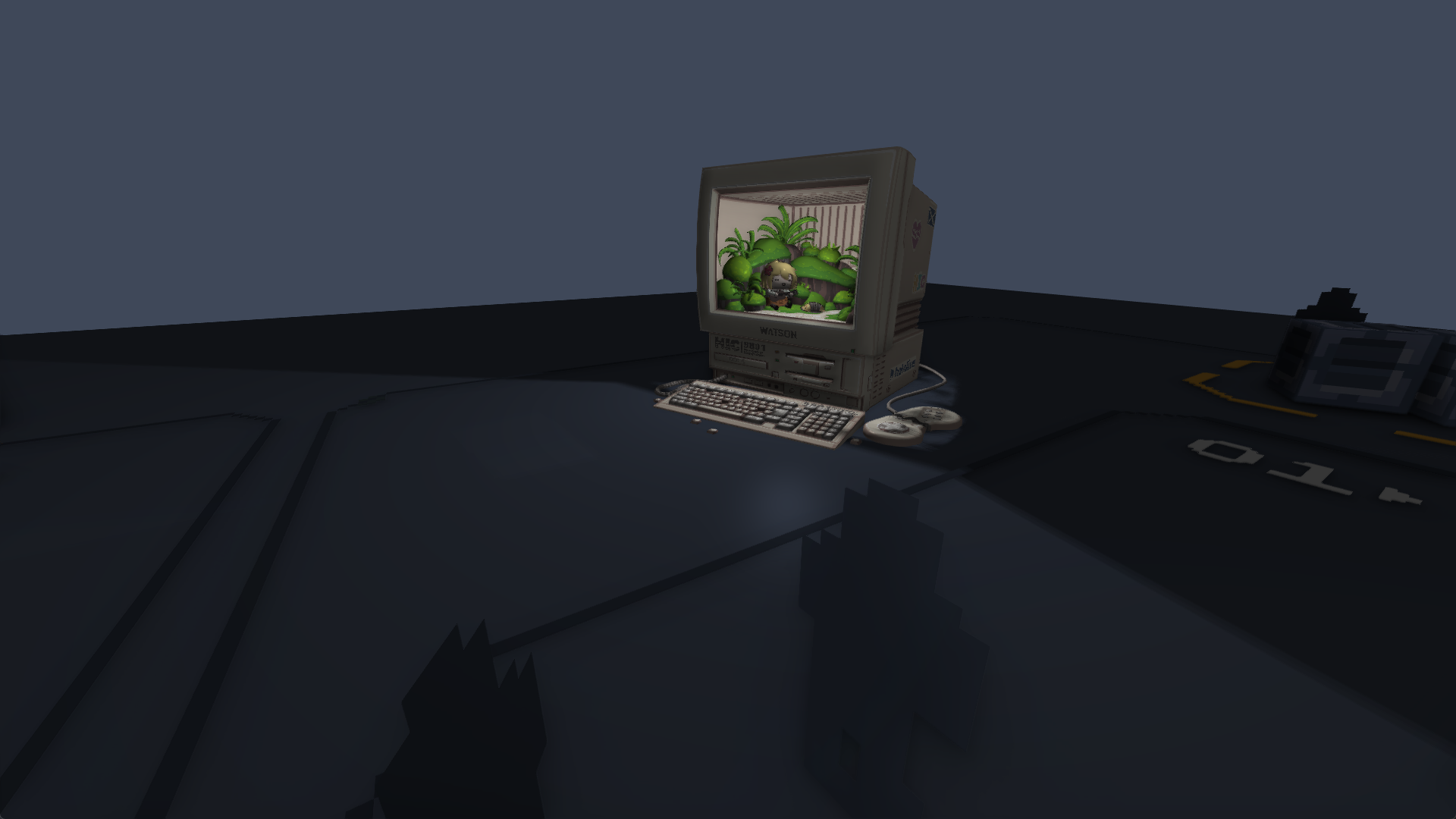
Ambient Light
It is in charge of giving a minimum of light to the scene. In this light we can see the result of the SSAO.
// Int. Color Entity l_ambient = Light::AmbientLight3D(cm, .25f, vec3(1.f));
Nintendo Switch Port
Thanks to the collection of libraries provided by DevKitPro, the engine has been ported to Switch. After configuring the Makefile and compiling the different libraries with the ARM compiler used to compile the rest of the application, a functional executable ready to be used on Switch, with command integration, can be obtained.
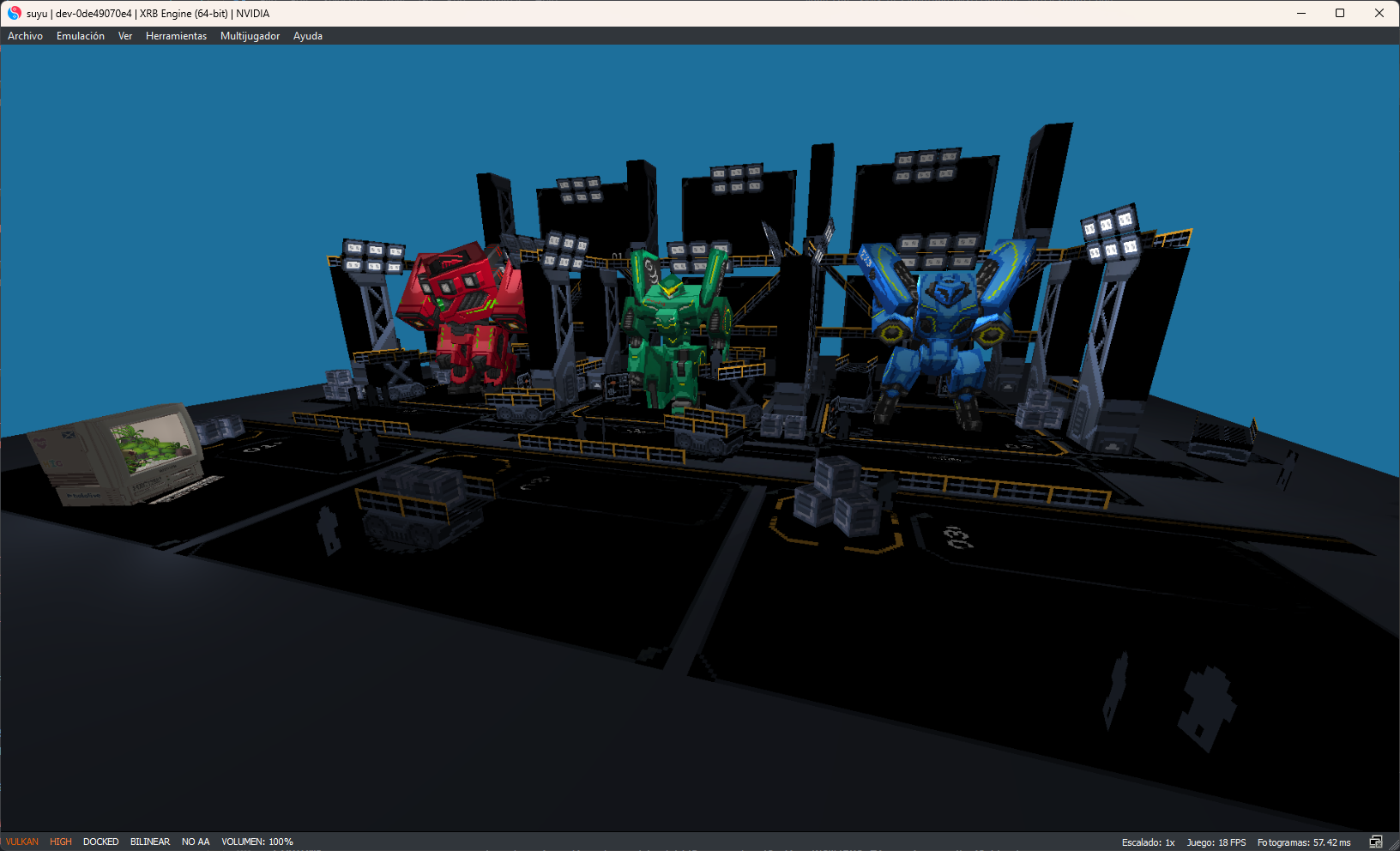